Coding, also known as programming, is the process of creating instructions that computers follow to perform tasks. Whether you’re interested in developing websites, building mobile apps, automating processes, or diving into data science, learning to code is an essential skill in today’s digital age. Coding is not just for computer science professionals anymore; it has become a valuable tool for anyone looking to understand and shape the technology that drives our world.
READ ALSO: Mastering Digital Transformation: Key Strategies for Businesses and Professionals
This comprehensive beginner’s guide will walk you through everything you need to know to get started with coding—from understanding the basics to choosing the right programming languages and learning resources. By the end of this guide, you’ll have a solid foundation to start your coding journey with confidence.
1. Understanding the Basics of Coding
What is Coding?
At its core, coding is the process of writing instructions that a computer can understand and execute. These instructions are written in programming languages, which serve as the medium through which humans communicate with machines. Coding is essential for creating software, websites, mobile applications, and even hardware programming. It allows you to automate tasks, solve complex problems, and create tools that can make life easier for others.
Coding can range from writing simple scripts that perform basic tasks to developing complex algorithms that power artificial intelligence and machine learning models. The possibilities are virtually limitless, making coding one of the most powerful skills you can learn.
Why Learn to Code?
Learning to code is more than just acquiring a new skill; it’s about opening up a world of opportunities. Coding enhances your problem-solving abilities and logical thinking, both of which are applicable in almost every profession. By learning to code, you can automate repetitive tasks, analyze large sets of data, and even bring your own digital ideas to life. Whether you’re a student, entrepreneur, or working professional, coding skills can significantly enhance your career prospects.
In today’s job market, coding is highly sought after. Employers across various industries look for individuals who can write code, regardless of whether they work in technology. For example, knowing how to code can give you an edge in fields like finance, marketing, healthcare, and education, where data-driven decision-making and automation are becoming increasingly important.
The Benefits of Learning Coding
Aside from career opportunities, coding offers several personal benefits:
- Creativity: Coding allows you to create something from nothing. Whether it’s a website, a game, or a mobile app, you can bring your ideas to life through code.
- Problem-Solving: Coding teaches you how to break down complex problems into manageable parts. This skill is invaluable in both your professional and personal life.
- Flexibility: Once you learn to code, you can work from anywhere. Many coding jobs offer remote work options, giving you the freedom to work on your own terms.
- Continuous Learning: The field of coding is always evolving, with new languages, frameworks, and technologies emerging regularly. This means you’ll always have something new to learn, keeping your skills sharp and relevant.
2. Choosing the Right Programming Language
Popular Programming Languages for Beginners
When you’re new to coding, choosing the right programming language to start with can feel overwhelming. Different languages are better suited to different tasks, so it’s important to align your choice with your goals. Here are some of the most popular programming languages for beginners:
- Python: Python is widely regarded as one of the best programming languages for beginners due to its readability and simplicity. It’s used in web development, data science, artificial intelligence, and automation. Python’s syntax is clean and easy to understand, making it an excellent starting point for those new to coding.
- JavaScript: If you’re interested in web development, JavaScript is an essential language to learn. It’s the backbone of web development, enabling you to create interactive and dynamic websites. JavaScript works alongside HTML and CSS to create the user interfaces and functionalities of web pages. It’s also used for server-side programming, mobile app development, and game development.
- HTML/CSS: While HTML (Hypertext Markup Language) and CSS (Cascading Style Sheets) aren’t technically programming languages, they are essential for web development. HTML structures the content of web pages, while CSS controls the layout and design. Learning HTML and CSS is the first step for anyone looking to build websites or work in front-end development.
- Java: Java is a versatile and powerful language used for building mobile apps, particularly for Android, as well as large-scale enterprise applications. Java is known for its portability, meaning code written in Java can run on any device that supports the Java Virtual Machine (JVM). It’s a great language for those interested in object-oriented programming and developing cross-platform applications.
- Ruby: Ruby is a user-friendly language often praised for its simplicity and productivity. It’s commonly used for web development, particularly with the Ruby on Rails framework, which simplifies many aspects of coding. Ruby’s clean and readable syntax makes it a good choice for beginners who want to learn programming quickly.
Which Language Should You Start With?
Your choice of language depends on what you want to achieve. If you’re interested in general-purpose programming or data science, start with Python. Its simplicity makes it ideal for learning core programming concepts that are applicable across many languages. If web development is your goal, JavaScript, along with HTML and CSS, is essential. For mobile app development, particularly for Android, Java is a strong candidate. Ruby is great if you want to dive into web development with a language that emphasizes productivity and simplicity.
3. Setting Up Your Coding Environment
Installing an IDE or Text Editor
To write and run your code, you’ll need a code editor or an Integrated Development Environment (IDE). A good coding environment is crucial for efficient programming, as it provides tools and features that make writing, testing, and debugging code easier. Here are some popular options:
- Visual Studio Code (VS Code): VS Code is a lightweight yet powerful text editor that supports a wide range of programming languages. It offers features like syntax highlighting, code completion, debugging tools, and a vast library of extensions that can enhance your coding experience.
- PyCharm: PyCharm is an IDE specifically designed for Python development. It provides advanced features like intelligent code completion, error checking, and built-in tools for testing and debugging. PyCharm is available in both a free community edition and a paid professional edition with more features.
- Sublime Text: Sublime Text is a fast, customizable text editor that’s popular among developers. It supports many programming languages and offers features like multi-line editing, powerful search and replace, and a wide range of plugins to extend its functionality.
- Atom: Developed by GitHub, Atom is a free, open-source text editor that’s highly customizable. It’s known for its ease of use, built-in Git integration, and a rich ecosystem of plugins.
- Eclipse: Eclipse is a powerful IDE primarily used for Java development, though it also supports other languages through plugins. It offers a comprehensive set of tools for coding, testing, and debugging, making it a popular choice for large-scale projects.
Setting Up Your First Project
Once you’ve installed your coding environment, it’s time to set up your first project. Starting with a simple project will help you get comfortable with the coding environment and the basics of programming. Here’s how you can get started:
- Create a New Project: In your IDE or text editor, create a new project or file. If you’re learning Python, for example, you can create a file named
hello_world.py
. - Write Your First Code: Begin with a simple program, like printing “Hello, World!” to the console.
- Run Your Code: Depending on your coding environment, you can run your code directly from the editor. In most cases, there’s a ‘Run’ button or a shortcut (like
F5
in VS Code) that will execute your program and display the output. - Explore and Experiment: Don’t be afraid to modify the code and see what happens. Try changing the text, adding new lines of code, or experimenting with variables and functions. This hands-on approach will deepen your understanding and help you learn faster.
4. Learning the Fundamentals
Key Concepts to Understand
Before diving into more complex projects, it’s essential to grasp the fundamental concepts of coding. These core principles are the building blocks of all programming languages:
- Variables: Variables are containers that store data values. For example,
name = "John"
assigns the value “John” to the variablename
. Variables can hold different types of data, such as numbers, text, or more complex data structures. - Data Types: Different types of data are handled differently in code. Common data types include integers (whole numbers), floats (decimal numbers), strings (text), and booleans (true/false values). Understanding data types is crucial for manipulating and storing information effectively.
- Control Structures: Control structures like loops (
for
,while
) and conditionals (if
,else
) control the flow of your program. They allow you to execute certain parts of your code based on conditions or repeat actions multiple times. - Functions: Functions are blocks of code that perform a specific task and can be reused throughout your program. For example, you might create a function to calculate the sum of two numbers and then call that function whenever you need to perform that calculation.
- Debugging: Debugging is the process of identifying and fixing errors in your code. Learning how to debug effectively is a critical skill, as it helps you troubleshoot issues and improve the quality of your code.
Practice Makes Perfect
The best way to learn coding is through consistent practice. Start with simple exercises that reinforce these concepts, such as:
- Basic Calculations: Write a program that performs basic arithmetic operations (addition, subtraction, multiplication, division) and prints the results.
- Simple Game: Create a basic text-based game, like a number guessing game, where the program chooses a random number, and the user has to guess it.
- Data Manipulation: Practice working with different data types by creating a program that processes a list of names, sorts them alphabetically, and prints the sorted list.
These exercises will help solidify your understanding of fundamental coding concepts and build your confidence as you progress to more complex projects.
5. Resources to Continue Learning
Online Courses and Tutorials
There are countless resources available to help you continue your coding journey. Here are some popular platforms that offer high-quality courses and tutorials:
- Codecademy: Codecademy offers interactive coding lessons in various programming languages. It’s an excellent platform for beginners, with hands-on exercises and projects that reinforce learning.
- Coursera: Coursera provides courses from top universities and institutions on a wide range of coding topics. You can find beginner courses in Python, web development, and more, often taught by industry experts.
- edX: Similar to Coursera, edX offers courses from leading universities. You can find both introductory and advanced programming courses that cater to different learning needs.
- freeCodeCamp: freeCodeCamp is a nonprofit organization that offers free coding lessons, projects, and certifications. It’s a community-driven platform where you can learn by building real-world projects.
- YouTube: Many developers and educators offer free coding tutorials on YouTube. Channels like CS50, Traversy Media, and The Net Ninja provide high-quality content for beginners.
Books for In-Depth Learning
If you prefer learning from books, here are some highly recommended titles:
- “Python Crash Course” by Eric Matthes: A hands-on, project-based introduction to Python. It covers basic programming concepts and provides a solid foundation for beginners.
- “JavaScript: The Good Parts” by Douglas Crockford: A concise and insightful guide to JavaScript, focusing on the language’s most powerful features. It’s a great read for anyone looking to master JavaScript.
- “Eloquent JavaScript” by Marijn Haverbeke: A modern introduction to JavaScript, with a focus on writing elegant and efficient code. The book includes interactive examples and exercises.
- “Automate the Boring Stuff with Python” by Al Sweigart: This book teaches Python through practical examples that automate everyday tasks, making it a valuable resource for beginners.
Joining Coding Communities
Coding is more fun and rewarding when you’re part of a community. Joining online coding communities can provide you with support, inspiration, and opportunities to collaborate on projects. Here are some popular coding communities:
- GitHub: GitHub is a platform for hosting and sharing code. It’s also a great place to collaborate on open-source projects and learn from other developers’ work.
- Stack Overflow: Stack Overflow is a question-and-answer site for programmers. If you run into any coding challenges, chances are someone has already asked and answered a similar question here.
- Reddit (r/learnprogramming): The learn programming subreddit is a friendly community where beginners can ask questions, share resources, and get advice from more experienced programmers.
- Discord: Many coding communities have Discord servers where you can chat with other learners, share your progress, and participate in coding challenges.
Conclusion
Learning to code is a journey that requires patience, practice, and perseverance. By starting with the basics and gradually building up your skills, you can unlock a world of possibilities in technology and beyond.
READ ALSO: The Ultimate Guide to Emerging Technologies: What to Watch in the Next Decade
Whether you aim to develop your own apps, contribute to open-source projects, or advance your career, coding is a valuable skill that will serve you well in the digital age. With the right resources and a commitment to learning, you’ll be well on your way to becoming a proficient coder.
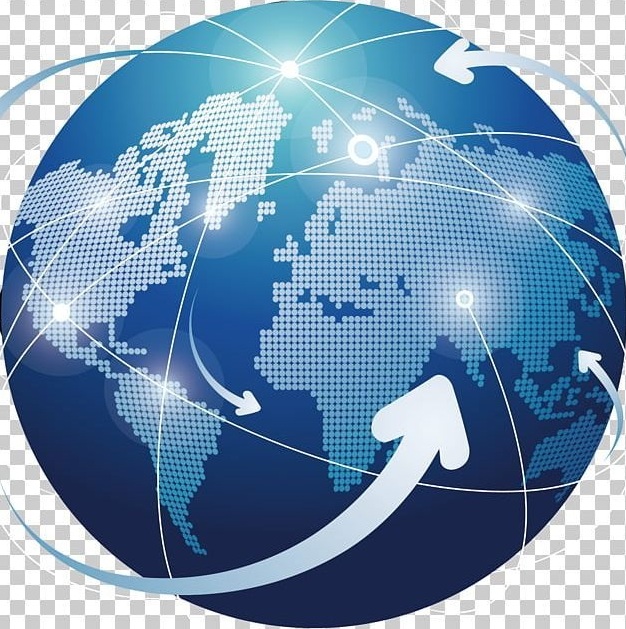
Tutorials Handbook is your trusted online resource blog for clear, insightful and comprehensive blog posts tailored to the unique needs of Kenyans. We cover a wide range of topics such as: Tutorials, Services, Business, Finance, Education, Jobs, Social Media and Technology, providing practical, informative and step-by-step blog posts on a day to day basis. We empower Kenyans by delivering accurate, helpful and relevant information.
FIND AND APPLY FOR THE LATEST JOBS IN KENYA TODAY